easygraphics.processing包¶
模仿Processing(http://processing.org)的动画模块。
from easygraphics import *
from easygraphics.processing import *
import math
x_spacing = 16 # How far apart should each horizontal location be spaced
theta = 0 # Start angle at 0
amplitude = 75 # Height of wave
period = 500 # How many pixels before the wave repeats
dx = (2 * math.pi / period) * x_spacing
def setup():
global w
set_size(640, 360)
translate(0, get_height() // 2)
set_background_color("black")
set_fill_color("white")
w = get_width() + 16 # Width of entire wave
def draw():
global theta
theta += 0.02
clear_device()
x = theta
for i in range(w // x_spacing):
y = math.sin(x) * amplitude
fill_circle(i * x_spacing, y, 8)
x += dx
run_app(globals())
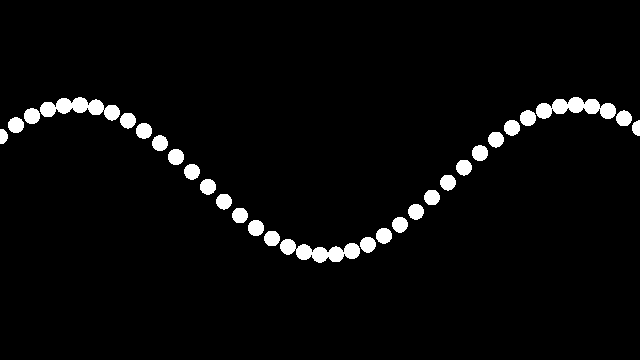
函数列表¶
待自定义(覆写)函数¶
draw () |
绘制一帧动画。 |
setup () |
设置Processing环境。 |
on_mouse_clicked () |
鼠标点击事件处理函数(方法)。 |
on_mouse_dragged () |
鼠标拖动事件处理函数(方法)。 |
on_mouse_pressed () |
按下鼠标按钮事件处理函数(方法)。 |
on_mouse_released () |
松开鼠标按钮事件处理函数(方法)。 |
on_mouse_wheel (e) |
鼠标滚轮事件处理函数(方法)。 |
控制与设置¶
full_screen () |
打开一个全屏幕大小的画布。 |
get_frame_rate () |
获取动画的帧率(fps)。 |
loop () |
开始动画循环。 |
mouse_pressed |
bool(x) -> bool |
mouse_x |
int([x]) -> integer int(x, base=10) -> integer |
mouse_y |
int([x]) -> integer int(x, base=10) -> integer |
noloop () |
停止动画循环。 |
prev_mouse_x |
int([x]) -> integer int(x, base=10) -> integer |
prev_mouse_y |
int([x]) -> integer int(x, base=10) -> integer |
redraw () |
调用一次draw()函数,来绘制动画的一帧。 |
set_frame_rate (fps) |
获取动画的帧率(fps)。 |
set_size (width, height) |
打开指定大小的画布(canvas)窗口。 |
函数¶
-
easygraphics.processing.
redraw
()¶ 调用一次draw()函数,来绘制动画的一帧。
不要自定义(覆写)该函数!
-
easygraphics.processing.
loop
()¶ 开始动画循环。
-
easygraphics.processing.
noloop
()¶ 停止动画循环。
-
easygraphics.processing.
run_app
(_globals)¶ 运行Processing程序。
参数: _globals – python的全局变量字典
-
easygraphics.processing.
set_size
(width: int, height: int)¶ 打开指定大小的画布(canvas)窗口。
参数: - width – 画布的宽度
- height – 画布的高度
-
easygraphics.processing.
full_screen
()¶ 打开一个全屏幕大小的画布。
-
easygraphics.processing.
draw
()¶ 绘制一帧动画。
你应该在程序中重新定义该函数。
返回:
-
easygraphics.processing.
setup
()¶ 设置Processing环境。
你应该在程序中重新定义该函数。
-
easygraphics.processing.
set_frame_rate
(fps: int)¶ 获取动画的帧率(fps)。
参数: fps – 帧率
-
easygraphics.processing.
get_frame_rate
() → int¶ 获取动画的帧率(fps)。
返回: 帧率
-
easygraphics.processing.
on_mouse_wheel
(e: PyQt5.QtGui.QWheelEvent)¶ 鼠标滚轮事件处理函数(方法)。
你应该在程序中重新定义该函数,以处理鼠标滚轮事件。
-
easygraphics.processing.
on_mouse_dragged
()¶ 鼠标拖动事件处理函数(方法)。
你应该在程序中重新定义该函数,以处理鼠标拖动事件。
-
easygraphics.processing.
on_mouse_released
()¶ 松开鼠标按钮事件处理函数(方法)。
你应该在程序中重新定义该函数,以处理松开鼠标按钮事件。
-
easygraphics.processing.
on_mouse_pressed
()¶ 按下鼠标按钮事件处理函数(方法)。
你应该在程序中重新定义该函数,以处理按下鼠标按钮事件。
-
easygraphics.processing.
on_mouse_clicked
()¶ 鼠标点击事件处理函数(方法)。
你应该在程序中重新定义该函数,以处理鼠标点击事件。
-
class
easygraphics.processing.
ProcessingWidget
(*args, auto_start=True, **kwargs)¶ 仿Processing窗口组件。
-
draw
()¶ 绘制一帧动画。
你应该覆写该方法。
-
full_screen
()¶ 将画布设置为全屏幕大小。
-
get_canvas
() → easygraphics.image.Image¶ 获取画布图片对象
返回: 图标图片对象
-
get_frame_rate
() → int¶ 获取动画的帧率(fps)。
返回: 帧率
-
keyPressEvent
(self, QKeyEvent)¶
-
loop
()¶ 开始动画循环。
-
mouseMoveEvent
(self, QMouseEvent)¶
-
mousePressEvent
(self, QMouseEvent)¶
-
mouseReleaseEvent
(self, QMouseEvent)¶
-
noloop
()¶ 停止动画循环。
-
on_mouse_clicked
()¶ 鼠标点击事件处理函数(方法)。
你可以覆写它,以处理鼠标点击事件。
-
on_mouse_dragged
()¶ 鼠标拖动事件处理函数(方法)。
你可以覆写它,以处理鼠标拖动事件。
-
on_mouse_pressed
()¶ 按下鼠标按钮事件处理函数(方法)。
你可以覆写它,以处理按下鼠标按钮事件。
-
on_mouse_released
()¶ 松开鼠标按钮事件处理函数(方法)。
你可以覆写它,以处理松开鼠标按钮事件。
-
on_mouse_wheel
(e: PyQt5.QtGui.QWheelEvent)¶ 鼠标滚轮事件处理函数(方法)。
你可以覆写它,以处理鼠标滚轮事件。
-
paintEvent
(self, QPaintEvent)¶
-
redraw
()¶ 调用一次draw()函数,来绘制动画的一帧。
-
set_frame_rate
(fps)¶ 获取动画的帧率(fps)。
参数: fps – 帧率
-
set_size
(width: int, height: int)¶ 设置画布大小。
参数: - width – 画布的宽度。
- height – 画布的高度。
-
setup
()¶ 设置动画环境。
你应该覆写该方法。
-
start
()¶ 手动开始准备动画环境,开始动画循环。
-
wheelEvent
(self, QWheelEvent)¶
-